Angular Framework Course
ANGULAR PROGRAMMING
JavaScript has become a cornerstone in modern web development. With its ability to handle complex interactivity and dynamic content, JavaScript paved the way for robust frameworks like Angular, which make web application development easier and more efficient. At Prolytics, our Angular course focuses on equipping students with the skills necessary to build scalable, high-performance applications.
Angular was designed to address common challenges faced by developers when working with JavaScript alone. It provides a well-defined architecture, two-way data binding, and a powerful dependency injection system, making it ideal for building modern, single-page applications.
What will you learn
-
Angular Core Concepts
You will learn key Angular concepts such as components, templates, directives, and services, giving you a solid foundation in building web applications.
-
Angular CLI Mastery
Understand how to efficiently create, develop, and maintain Angular applications using Angular CLI.
-
Reactive Forms and Validation
Learn how to build dynamic and reactive forms with custom validation rules for better user input handling.
-
State Management
Gain expertise in managing state effectively using Angular services and libraries like NgRx.
By completing this course, you will develop expertise in the following areas:
- Angular architecture and components
- Data binding and dependency injection
- Template-driven and reactive forms
- Creating reusable services and directives
- Developing Single Page Applications (SPAs)
- Routing and navigation
- HTTP client and RESTful APIs integration
- Animations in Angular
- State management with NgRx
- Application deployment and optimization
Lessons
- Duration
- 45 Days
Learn about Angular, its history, and why it is essential for modern web development. | |
Set up your development environment for Angular using Angular CLI. |
Understand the structure of Angular applications using components and templates. | |
Learn to create reusable components for efficient code management. |
Master built-in directives such as *ngIf and *ngFor for dynamic content rendering. | |
Create custom directives and pipes to extend Angular’s capabilities. |
Learn one-way and two-way data binding to create interactive applications. | |
Understand dependency injection and how Angular manages services. |
Implement routing to create single-page applications with multiple views. | |
Learn how to pass parameters and manage route guards for better navigation control. |
Explore template-driven and reactive forms for collecting and validating user input. | |
Create custom form validators to enforce complex business rules. |
Learn to consume RESTful APIs using Angular HTTP client. | |
Understand how to work with Observables and RxJS for asynchronous data streams. |
Manage application state effectively using NgRx, a popular Angular state management library. | |
Implement actions, reducers, and selectors for better state control. |
Enhance the user experience by adding animations using Angular animation package. | |
Learn to create smooth transitions and effects for your web applications. |
Write unit tests for components, services, and directives using Jasmine and Karma. | |
Deploy your Angular application to production environments. |
Learn best practices for optimizing Angular applications for speed and performance. | |
Understand lazy loading, AOT compilation, and efficient change detection strategies. |
Learn to build installable and offline-capable PWAs using Angular. | |
Understand service workers and caching strategies for offline support. |
You Can Trust with Global Certification
At Prolytics Institute, our certifications are globally recognized, ensuring that your skills are validated by a trusted authority. Whether you're advancing in your current role or exploring new career opportunities, our credentials give you a competitive edge in today’s global job market.
Our certification programs are designed to meet international standards while catering to local industry needs. This ensures that your expertise is not only widely accepted but also practically applicable wherever you go.
Committed to preparing you for successful career placement and acing job interviews.
Our curriculum is thoughtfully crafted by senior angular specialists with extensive industry experience.
The course prioritizes cutting-edge technologies that are in high demand among top web design firms and global corporations.
A Personality Development program that enhances skills in professional communication, email writing, and effective interview techniques.
Engaging hands-on practice and real-world projects form a key part of the learning experience.
Learn directly from professionals who are currently active as developer in top IT companies.
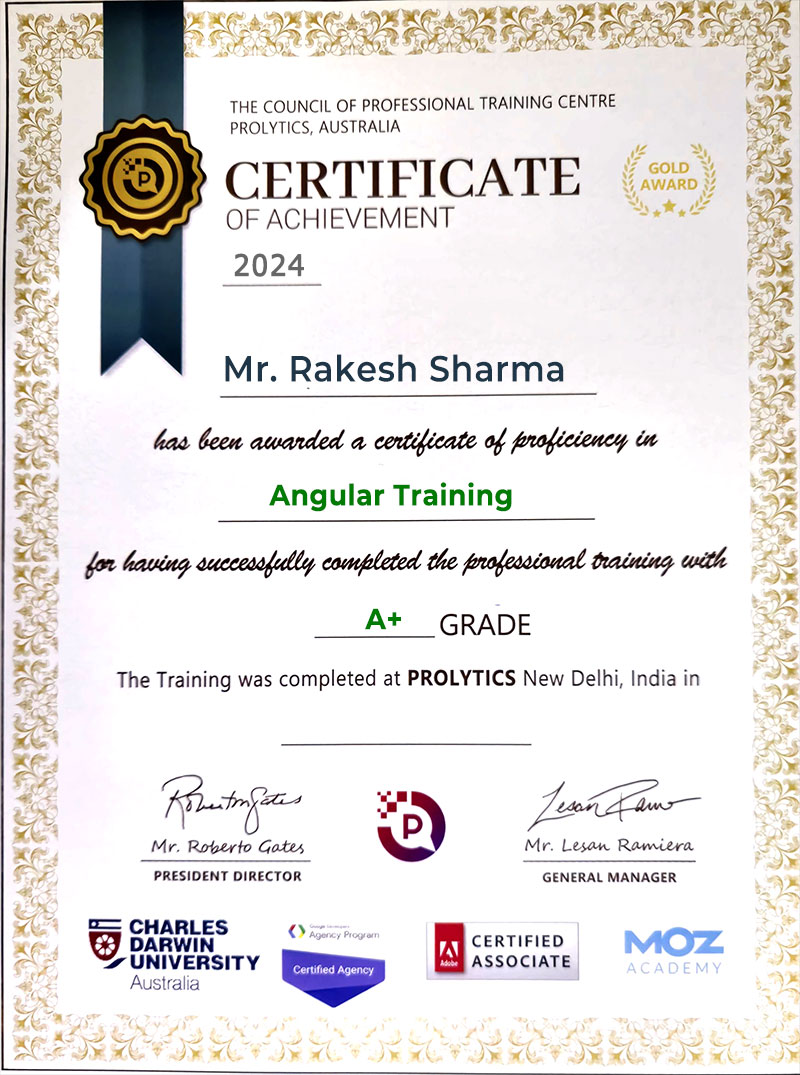
FAQs
General frequently asked questions for students.
Our instructors are experienced professionals actively working in the IT industry. They bring real-world insights, practical knowledge, and expertise to the classroom, enhancing the learning experience.
Yes, we offer dedicated job placement support to our graduates. This includes resume workshops, mock interviews, and direct connections with our industry partners to help students transition smoothly into their careers.
Prolytics provides both in-person and online classes to cater to various learning preferences. Our online classes are live and interactive, allowing students to engage directly with instructors and peers.
Absolutely! We prioritize hands-on learning through practical projects and assignments based on real-world scenarios. These projects help students gain practical experience and build a portfolio to showcase to potential employers.
Yes, upon successfully completing a course, students receive a certificate from Prolytics, recognized by industry partners and highly valued in the job market.
Prolytics has a flexible refund policy. Students who need to withdraw may be eligible for a partial refund, depending on the timing and course structure. Please contact our support team for detailed information on refunds.
To enroll, visit our website and register the enrollment form for your desired course. You can also reach out to our admissions team for guidance on course selection and the enrollment process.
Students Reviews
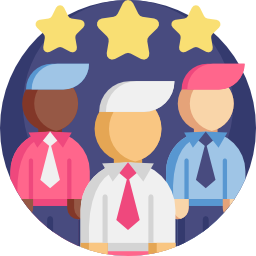
Right from a basic introduction to Angular JS to its more advanced concepts and techniques, the Prolytics team helped me become proficient in this method of programming. Thank you guys!
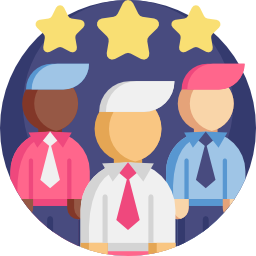
My favorite part of the Angular JS course taught at Prolytics is that it covers so much in such a short span of time! And the material provided is very comprehensive.
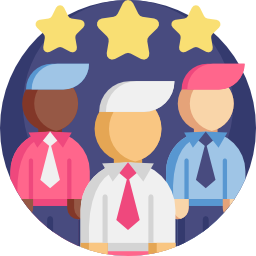
I wanted a course on Angular JS that taught everything there is to learn about it and the Prolytics course did just that. That too, at a very fantastic price!