ASP.NET
ASP.NET
It is blazingly fast, cross-platform, and cloud-ready. Microsoft has done groundbreaking redesigns to ASP.NET Core and so lots of new concepts were introduced along with it. In this course, we’ll take a look at them while creating a website from scratch.
In this course, you’ll learn how to do the basic setup for the project, and then we’ll take a dive into the concept of the MVC pattern. You’ll learn about models, views, and controllers in detail. You will see the new concept of middleware and it will be demystified. Dependency Injection (DI) is now native to ASP.NET Core MVC and comes already wired up. You will learn to use DI with ease. Routing is central to ASP.NET Core MVC and there is a dedicated section for learning all that you need to know so that you can create effective routing for your site. We’ll discuss the Razor syntax for UI programming and work with the Entity Framework core and create a Database in the SQLLocalDb which is the new developer friendly Database server around in the block.
What will you learn
-
Understanding core concepts
Get to know the concepts of ASP .NET MVC and build a new static web page using HTML, CSS, and jQuery.
-
ASP.NET Lightning Experience
Build and run your first application.
-
Setting up website
Create Mock data for your website and set up DI Containers.
-
Basics of Framework
Explore configuration of Entity Framework Core.
-
MVC Application
Construct a model for an ASP .NET Core MVC application.
-
MVC Routing
Understand the importance of Routing in MVC.
-
Views & Razor
Get an in-depth understanding of Views and Razor Syntax.
Lessons
- 40 Lectures
- 12:46:32
- Dot Net framework Using ASP.net and C# Reviews
- OOPs concept using C#
- Communication and Collaborations
- Controls in ASP.Net & ASP. Net objects
- Master pages
- Themes
- Skins
- User control
- Ajax Control Tool kit
- Validation controls & security Navigation tools
- Database concepts
- Data controls
- Classes
- Objects
- DLL's
- Publishing & Deploying Web App
- Membership service
- Advance concepts – LinQ
- Projects
Students Reviews
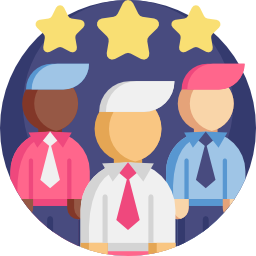
I did web ASP.NET course from Prolytics and this was my best experience.